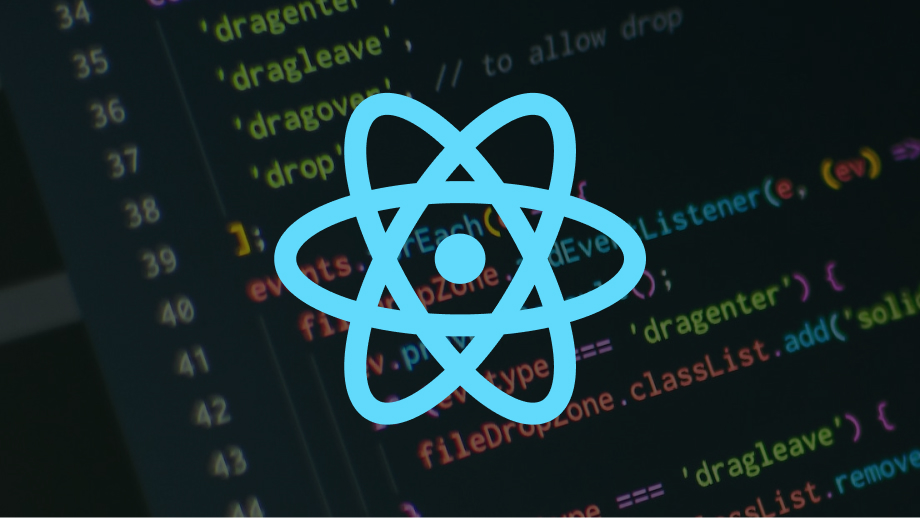
Inheriting Methods & Properties
When creating a new Class you can inherit and use the properties and methods from the parent Class with calling super(); in your constructor();
Class Human(){
constructor(){
this.race = "Human";
}
printRace(){console.log(this.race)}
}
Class Person extends Human {
constructor(){
this.name = "Jasmin";
super(); // <= this executes the initializaton of the parent methods and properties
}
printName(){
console.log(this.name)
}
me = new Person();
me.printName() // "Jasmin"
me.printRace() // "Human"
}
Classes, Properties & Methods
Properties are like variables attached to Classes & Objects
- in ES6 you have to define properties within the constructor function
constructor(){
this.prop = "something";
}
In ES7 you can define it without constructor via myProperty = "Something"
Spread & Rest operator “…”
Spread & Rest operator is simple (three-dot) function that is used to split up array elements or object properties (SPREAD) or merge a list of function arguments into an array (REST)
const newArray = [...oldArray,5,6,7]; // spread
const newObject = { // Spread
...oldObject,
propNickName: "Mickey"
}
function newFunction(...args){ //rest
return args.sort();
}
Equality test of a property
When comparing the variables, properties or filtering an array, we often use == and ===, the main and only difference is:
==
compares the value of the argument and===
compares value & type of the argument
Destructuring Arrays & Objects
Easily extract array values or object properties to store them in variables
// Array Destructuring
[greeting, name] = myArray // myArray = ["Hello","Mickey"]
console.log(greeting) // Hello
console.log(name) // Mickey
//Object Destructuring
myObj = {
name: "Jasmin",
age: 32
}
{name} = myObj;
console.log(name) // Jasmin
console.log(age) // undefined (since we did not extract age, only name
Reference & Primitive types
Important to notice, when copying the value of primitive types (boolean, string, number…) you will strictly copy their value. If you copy an Array or Object you will only refer to the existing object. This can give you a headache in React development sometimes, that is why “real” copying for Arrays and Objects is done with a spread operator. For example:
// Primitive types: String, number, boolean
yourAge = 34;
myAge = yourAge;
console.log(myAge) // 34
yourAge = 55; //redefine yourAge
console.log(myAge) // 34 <= stays as is
// Objects or Arrays:
cat = {
name: "Jasmin"
}
dog = cat;
console.log(dog.name) // Jasmin
//change value of cat.name changes the name of the dog as well
cat.name = "Manuela"
console.log(dog.name) // Manuela
In order to really copy your object properties, you can use the spread operator
cat = {name: "Jasmin"}
dog = {...cat}
console.log(dog.name) // Jasmin
cat.name = "Manuela";
console.log(dog.name) // Jasmin
Array Functions
Those functions like map() , find()
, filter()
or similar, are often used in react components. Check the for more information check the Mozilla Docs